Anubhav's String Length Solution
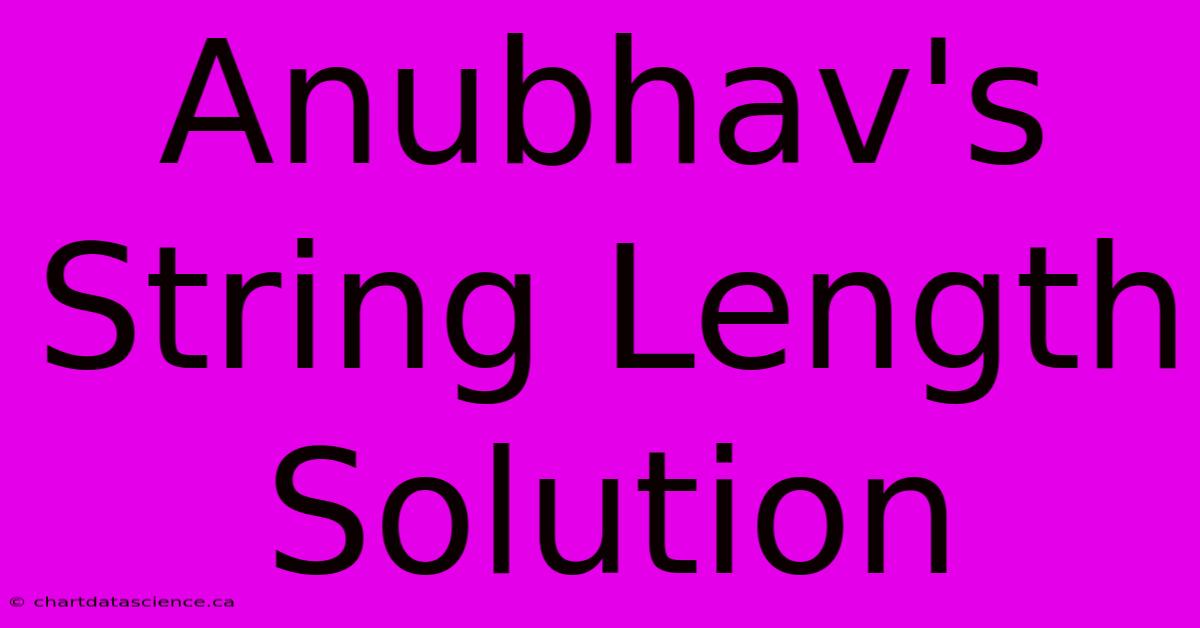
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit My Website. Don't miss out!
Table of Contents
Anubhav's String Length Solution: A Deep Dive
Anubhav's String Length Solution isn't a single, formally defined algorithm. Instead, it refers to a common approach to determining the length of a string, often encountered in introductory programming courses and coding challenges. It highlights several crucial concepts in string manipulation and programming fundamentals. This article delves into the core principles behind Anubhav's (or similar) approaches, exploring different implementations and optimizations.
Understanding the Problem: Finding String Length
The fundamental problem is straightforward: given a string, determine its length (the number of characters it contains). This seemingly simple task reveals important aspects of how strings are stored and accessed in computer memory.
Anubhav's Approach: Iterative Counting
Anubhav's solution, in its essence, likely employs an iterative approach. This means it systematically traverses the string, character by character, incrementing a counter for each character encountered. The process continues until the end of the string is reached, at which point the counter holds the string's length.
Implementation in Python:
def string_length(input_string):
"""
Calculates the length of a string iteratively.
"""
length = 0
for _ in input_string:
length += 1
return length
# Example usage
my_string = "Hello, world!"
length = string_length(my_string)
print(f"The length of '{my_string}' is: {length}")
Implementation in C++:
#include
#include
int stringLength(const std::string& str) {
int length = 0;
for (char c : str) {
length++;
}
return length;
}
int main() {
std::string myString = "Hello, world!";
int len = stringLength(myString);
std::cout << "The length of '" << myString << "' is: " << len << std::endl;
return 0;
}
Built-in Functions: A More Efficient Solution
While Anubhav's iterative method is conceptually clear, most programming languages provide built-in functions that are significantly more efficient. These functions are typically optimized at a lower level, leveraging the language's internal knowledge of string storage.
Python: len()
function. This is the preferred and most efficient way to find the length of a string in Python.
my_string = "Hello, world!"
length = len(my_string) #Direct and efficient
print(f"The length of '{my_string}' is: {length}")
C++: string.length()
or string.size()
methods. Similar to Python's len()
, these are the recommended approaches for C++.
#include
#include
int main() {
std::string myString = "Hello, world!";
int len = myString.length(); // Preferred method
std::cout << "The length of '" << myString << "' is: " << len << std::endl;
return 0;
}
Advantages and Disadvantages
Anubhav's Iterative Approach:
- Advantages: Excellent for educational purposes; demonstrates fundamental looping concepts.
- Disadvantages: Less efficient than built-in functions; potentially slower for very large strings.
Built-in Functions:
- Advantages: Highly optimized; generally faster and more efficient; simpler code.
- Disadvantages: May obscure the underlying mechanics of string length calculation for beginners.
Conclusion
Anubhav's String Length Solution serves as a valuable introduction to string manipulation. While the iterative approach offers educational benefits, using built-in functions is always recommended for practical applications due to their superior performance and simplicity. Understanding both approaches provides a complete understanding of the problem and its solutions. Remember to choose the method best suited to your needs, prioritizing efficiency and code readability.
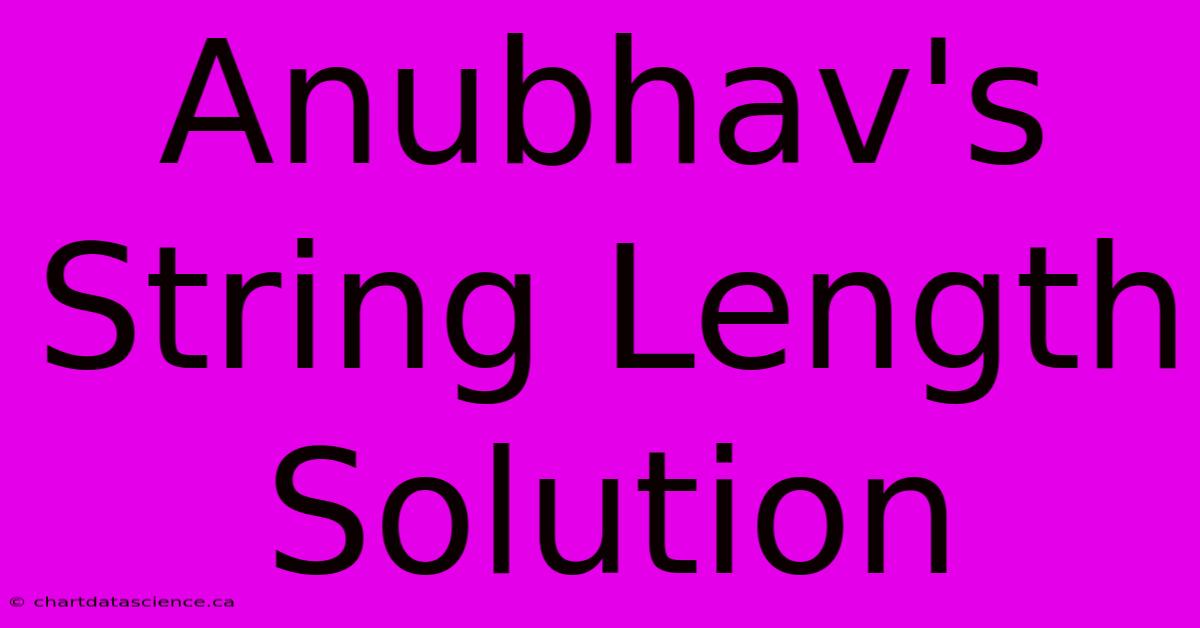
Thank you for visiting our website wich cover about Anubhav's String Length Solution. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
15 Year Old Girl Identified In Wisconsin School Shooting | Dec 17, 2024 |
At Least 6 Dead In Vanuatu Earthquake | Dec 17, 2024 |
Kiefer Sherwood Key Offensive Contributor | Dec 17, 2024 |
Cloverdale Langley City Conservatives Triumph | Dec 17, 2024 |
Police Identify Alleged Wisconsin School Shooter 15 | Dec 17, 2024 |