HSC Star Solves String Length
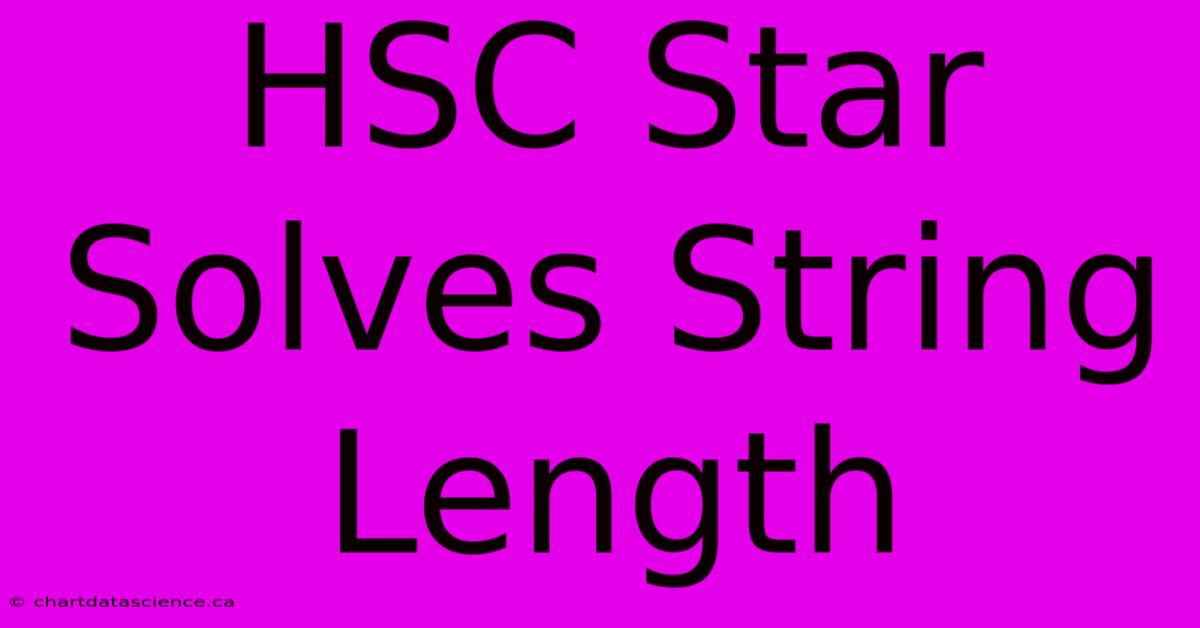
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit My Website. Don't miss out!
Table of Contents
HSC Star Solves String Length: A Comprehensive Guide
The HSC (Higher Secondary Certificate) exam often features challenging programming problems. One such problem, frequently encountered, involves determining the length of a string. While seemingly straightforward, efficient and elegant solutions are crucial for maximizing marks and demonstrating strong programming skills. This article provides a comprehensive guide to solving string length problems, focusing on clarity, efficiency, and best practices applicable to the HSC exam context.
Understanding the Problem
The core task is to write a program that accurately calculates the length of a given string. The input will be a string, and the output should be its length (the number of characters). This might seem trivial, but the challenge often lies in handling different input scenarios and optimizing for speed and memory efficiency, especially when dealing with potentially large strings.
Common Approaches and Their Efficiency
Several methods can determine string length. Let's analyze the most common approaches, focusing on their suitability for the HSC exam:
1. Using Built-in Functions (Most Efficient)
Most programming languages provide built-in functions specifically designed for this purpose. For example:
- Python:
len()
function. This is the most efficient and recommended approach in Python. - C++:
.length()
method forstd::string
objects. This is the standard and efficient way in C++. - Java:
.length()
method forString
objects. Similar to C++, this is the preferred method.
Example (Python):
my_string = "Hello, World!"
string_length = len(my_string)
print(f"The length of the string is: {string_length}")
This method is highly recommended for its simplicity, efficiency, and readability. It's the best approach for the HSC exam as it demonstrates understanding of standard library functions and avoids unnecessary complexity.
2. Iterative Approach (Less Efficient)
A less efficient but conceptually simpler approach involves iterating through the string and counting characters. This method is less preferred for the HSC exam due to its lower efficiency compared to built-in functions. However, understanding it can be helpful for foundational knowledge.
Example (Python):
my_string = "Hello, World!"
string_length = 0
for char in my_string:
string_length += 1
print(f"The length of the string is: {string_length}")
While functional, this approach is slower, especially for very long strings. It's generally not the best choice for HSC exams unless the question specifically requires this method to be used.
3. Recursive Approach (Generally Inefficient)
A recursive approach is generally not recommended for this problem in the context of the HSC exam. It adds unnecessary complexity and is significantly less efficient than the built-in function or iterative methods. Recursion can lead to stack overflow errors with very long strings.
Handling Special Cases and Edge Conditions
While built-in functions often handle edge cases automatically, it's good practice to consider them:
- Empty String: The length of an empty string ("") should be 0. Built-in functions generally handle this correctly.
- Strings with Special Characters: The length should include special characters (e.g., spaces, punctuation). Built-in functions correctly account for all characters.
- Unicode Characters: Modern languages handle Unicode characters effectively. The length should include all characters regardless of their encoding.
Best Practices for HSC Exams
- Choose the most efficient method: Use built-in functions (
len()
in Python,.length()
in C++/Java) for optimal performance and readability. - Write clean and well-commented code: Clearly document your code's purpose and logic. This is crucial for HSC marking.
- Handle edge cases: While built-in functions usually handle these, demonstrating awareness is beneficial.
- Test your code thoroughly: Test with various inputs, including empty strings, strings with special characters, and long strings.
By following these guidelines, you can confidently tackle string length problems in your HSC exams, showcasing your programming proficiency and achieving high marks. Remember, choosing the most efficient and readable solution is key to success.
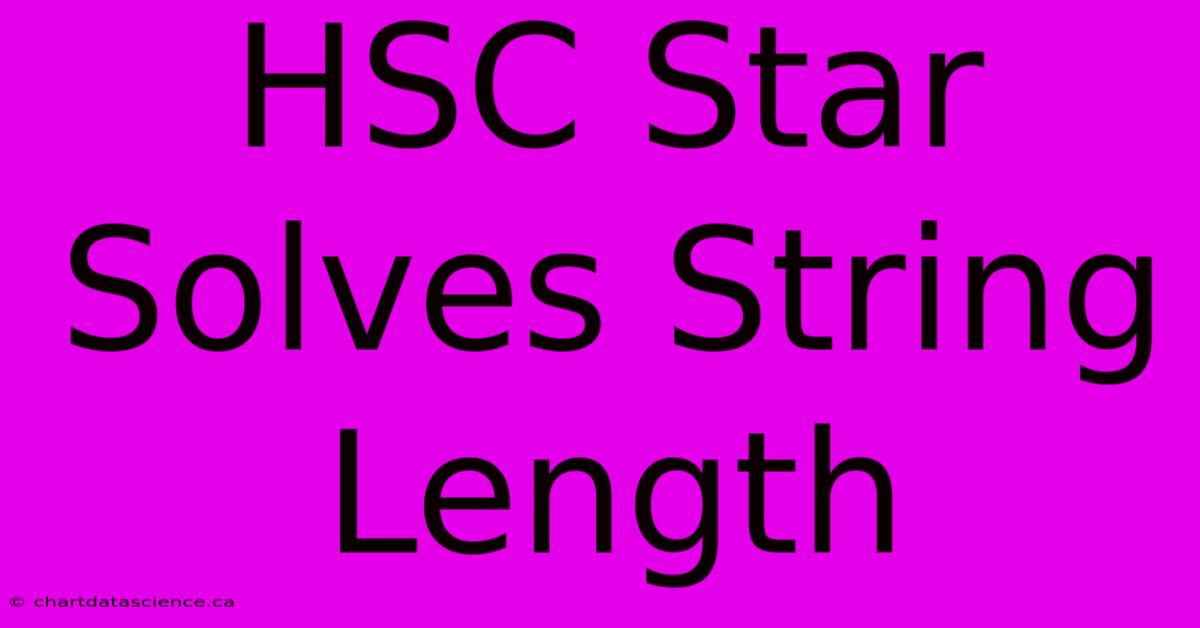
Thank you for visiting our website wich cover about HSC Star Solves String Length. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
Canada Post Resuming Impact On You | Dec 17, 2024 |
Vanuatu Hit By Deadly Earthquake | Dec 17, 2024 |
Vanuatu Earthquake 6 Dead | Dec 17, 2024 |
Prince Andrew Spy Link Controversy | Dec 17, 2024 |
Reflecting On Mishal Husains Radio 4 Legacy | Dec 17, 2024 |