String Length: An HSC Approach
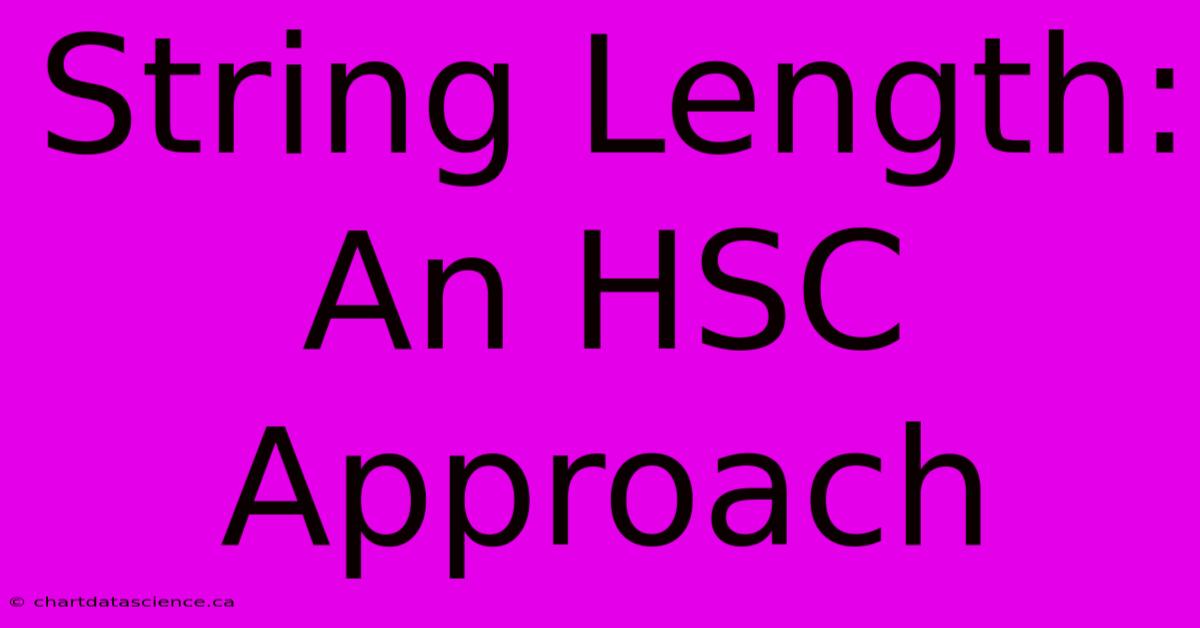
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit My Website. Don't miss out!
Table of Contents
String Length: An HSC Approach
The concept of string length is fundamental in computer science, and a crucial topic for students preparing for the Higher School Certificate (HSC). Understanding string length, and how to manipulate it, is essential for tackling various programming challenges. This article provides a comprehensive overview of string length, focusing on its application within the HSC context.
What is String Length?
In programming, a string is a sequence of characters. The string length refers to the total number of characters within that string. This includes letters, numbers, spaces, and punctuation marks – every character contributes to the overall length.
For example:
"Hello"
has a length of 5." HSC"
has a length of 4 (including the leading space).""
(an empty string) has a length of 0.
Determining String Length in Different Programming Languages
The method for determining string length varies slightly depending on the programming language used. However, the core concept remains consistent.
Python
Python provides a built-in function, len()
, to easily obtain the length of a string:
my_string = "Hello, world!"
string_length = len(my_string)
print(string_length) # Output: 13
Java
In Java, the length()
method is used with String
objects:
String myString = "Hello, world!";
int stringLength = myString.length();
System.out.println(stringLength); // Output: 13
C++
C++ uses the length()
method (or size()
) for strings (using the <string>
library):
#include
#include
int main() {
std::string myString = "Hello, world!";
int stringLength = myString.length();
std::cout << stringLength << std::endl; // Output: 13
return 0;
}
Practical Applications in HSC Programming
Understanding string length is crucial for a variety of HSC programming tasks, including:
1. Input Validation
Many programs require user input. Checking the length of the input string ensures the data meets specific criteria. For instance, a password might need to be at least 8 characters long.
2. String Manipulation
String length is often used in algorithms that manipulate strings. For example, you might need to:
- Truncate strings: Shorten strings to a specific length.
- Pad strings: Add characters to the beginning or end of a string to reach a desired length.
- Center strings: Align text within a fixed-width field.
3. Data Processing
String length plays a vital role in processing textual data. This could involve analyzing text files, counting words, or extracting specific information from strings.
Common HSC Exam Questions Related to String Length
HSC exams often test students' understanding of string length through various question types. These might include:
- Coding questions: Write a program that calculates and displays the length of a string.
- Problem-solving questions: Determine the length of a string given specific conditions.
- Algorithmic questions: Design algorithms that use string length for string manipulation tasks.
Mastering String Length for HSC Success
To excel in the HSC, focus on these key aspects of string length:
- Understand the core concept: Clearly grasp the meaning and significance of string length.
- Master the language-specific methods: Become proficient in using functions or methods to determine string length in your chosen programming language(s).
- Practice, practice, practice: Solve numerous programming problems that involve string length manipulation.
- Review past papers: Analyze past HSC exam questions to understand common question styles and challenges.
By mastering string length, you will be well-equipped to tackle a wide range of programming problems and achieve success in your HSC computer science examinations. Remember to consult your course materials and seek assistance from your teachers or tutors if you encounter difficulties.
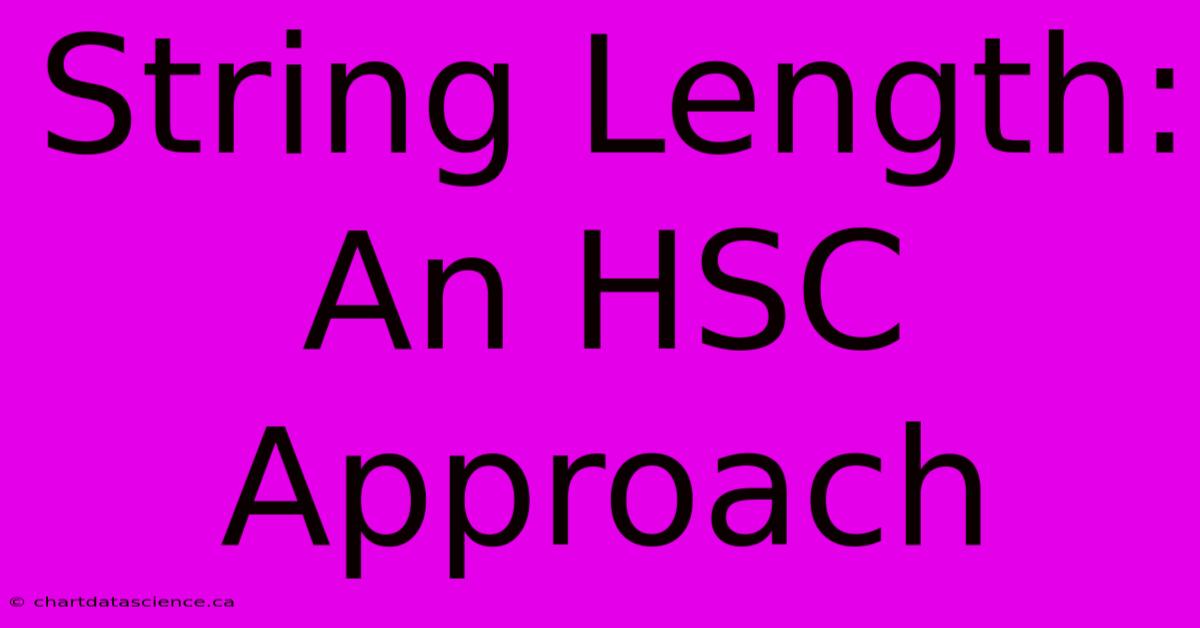
Thank you for visiting our website wich cover about String Length: An HSC Approach. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
Yang Tengbo Link To Prince Andrew | Dec 17, 2024 |
Introducing Taima Seahawks Mascot | Dec 17, 2024 |
Randy Moss Cancer Battle Vikings Tribute | Dec 17, 2024 |
Abc Names Hugh Marks Managing Director | Dec 17, 2024 |
Premier League West Ham Bournemouth Result | Dec 17, 2024 |